【CodeIgniter4】Web APIを作成する方法
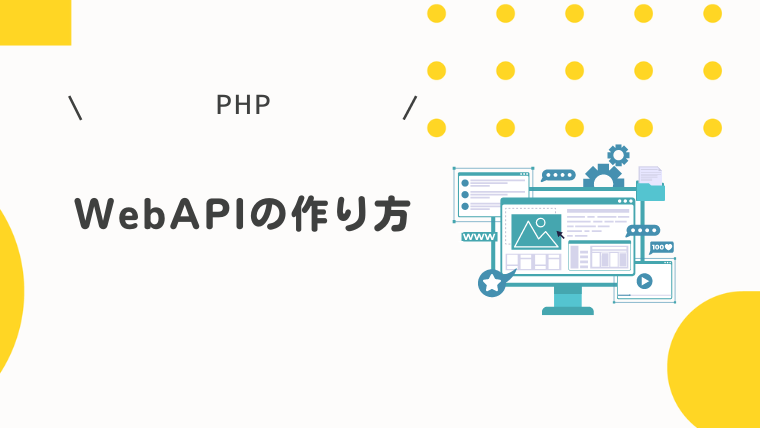
はい、hebiです。
CodeIgniter4でWebAPIを作成したいと思います。
フロントからはjQueryのajaxを利用して実行します。ajaxについては以下の記事を参考にどうぞう!
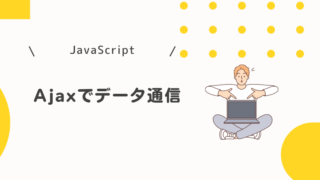
Web APIの作成
フロントからリクエストを受け付けるためのコントローラを作成しましょう。
ユーザー情報を取得するためのUserクラスとget_userメソッドを作成します。
<?php
namespace App\Controllers;
class User extends BaseController
{
public function get_user($user_id)
{
// user_idをもとにデータを取得する処理を書く
return $this->response->setStatusCode(200)->setJSON(['name' => 'hebi']);
}
}
HTTP Status 200(成功)を返却し、jsonでnameを返却するコントローラを作成しました。
app/Config/Routes.php ファイルで、GETリクエストを作成したUserコントローラのget_userにマッピングします。
これによりフロントからget_userを実行できるようになります。
<?php
use CodeIgniter\Router\RouteCollection;
/**
* @var RouteCollection $routes
*/
$routes->get('/', 'Home::index');
// ユーザ取得
$routes->get('user/(:num)', 'User::get_user/$1');
ユーザを取得するためにidを受け取るようにしているので、(:num)、$1を指定しています。
以上でサーバ側の実装完了です。
フロントからAPIを実行
作成したWebAPIをフロントから実行してみましょう。
ルーティングで設定したuserをurlに指定してGETメソッドでajaxを実行します。引数にはを123を指定してます。
function getUser(e) {
$.ajax({
url: 'index.php/user/123',
type: 'GET',
dataType: 'json',
success: function(response) {
appendAlert(response.name,'success')
},
error: function(xhr, status, error) {
if (xhr.status === 0) {
appendAlert('ネットワークエラーです。','warning')
} else if (xhr.status === 404) {
appendAlert('存在しません。','warning')
}else{
appendAlert('その他サーバーエラーです。','danger')
}
}
});
}
では実際に動かしてみましょう!
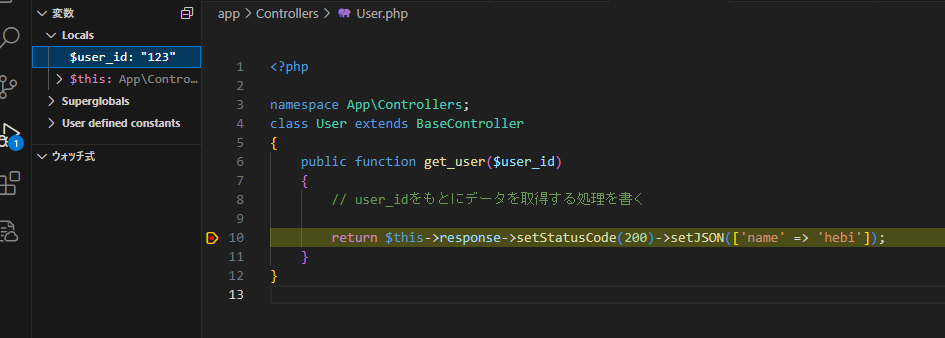
user_idに123が入り、get_userが正常に呼び出されました!
また、フロント側でhebiを受け取ることができました!
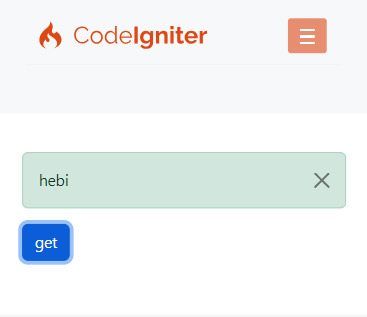
以上でフロント側の実装完了です。
いや、ちょまっっ!!index.php/user/123
のindex.phpはいらないんだけど!!と思いましたよね??
なぜかCodeIgniterの場合はindex.phpを付けないと404エラーになってしまいます。
index.phpをurlに含めなくするには設定が必要なのでやっていきましょう。
index.phpを含めない方法
$indexPathを”に設定
「app\Config\App.php」の$indexPageを’’にします。
// public string $indexPage = 'index.php';
public string $indexPage = '';
Apacheの設定ファイルを開く
以下のコマンドでApacheの設定ファイルを開きます。
sudo nano /etc/httpd/conf/httpd.conf
Apacheの設定ファイル変更
以下のように変更します。
#<Directory "/var/www/html/my_codeigniter_project/public">
# AllowOverride none
# Allow open access:
# Require all granted
#</Directory>
<Directory "/var/www/html/my_codeigniter_project">
AllowOverride All
Require all granted
</Directory>
AllowOverride Allを設定することで、.htaccessファイルが有効になり、Apacheの設定ファイルに直接ルーティングを追加することなく、リライトルールを使用してURLのリライトが可能になります。つまり、AllowOverride Allを使用すると、.htaccessファイル内のルーティング設定が有効になり、URLにindex.phpを含めずにCodeIgniterのコントローラやメソッドを直接指定できるようになります。
Apache再起動
Apacheを再起動します。
sudo systemctl restart httpd
index.phpなしで再実行
urlにindex.phpなしで指定してajaxを実行します。
function getUser(e) {
$.ajax({
url: '/user/123',
type: 'GET',
dataType: 'json',
success: function(response) {
appendAlert(response.name,'success')
},
error: function(xhr, status, error) {
if (xhr.status === 0) {
appendAlert('ネットワークエラーです。','warning')
} else if (xhr.status === 404) {
appendAlert('存在しません。','warning')
}else{
appendAlert('その他サーバーエラーです。','danger')
}
}
});
}
ちゃんとget_userが呼ばれることを確認できました!
index.phpなしで再実行
urlにindex.phpなしで指定してajaxを実行します。
function getUser(e) {
$.ajax({
url: '/user/123',
type: 'GET',
dataType: 'json',
success: function(response) {
appendAlert(response.name,'success')
},
error: function(xhr, status, error) {
if (xhr.status === 0) {
appendAlert('ネットワークエラーです。','warning')
} else if (xhr.status === 404) {
appendAlert('存在しません。','warning')
}else{
appendAlert('その他サーバーエラーです。','danger')
}
}
});
}
ちゃんとget_userが呼ばれることを確認できました!
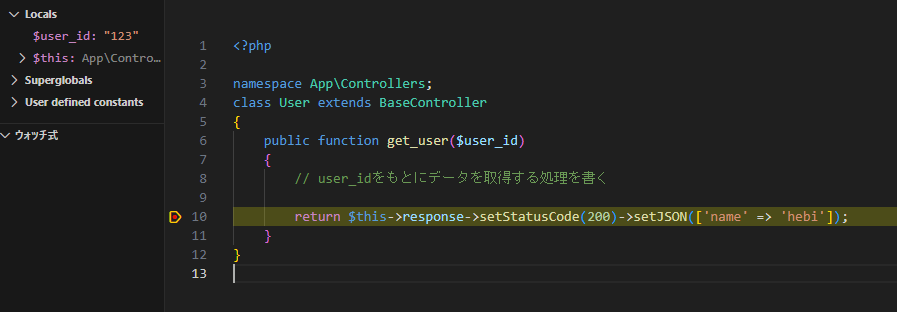
POSTリクエスト用のAPIを作成
POSTリクエストを利用したAPIも作成してみましょう。
コントローラにPOSTのAPIを追加
Userクラスにadd_user関数を追加します。
<?php
namespace App\Controllers;
class User extends BaseController
{
public function get_user($user_id)
{
// user_idをもとにデータを取得する処理を書く
return $this->response->setStatusCode(200)->setJSON(['name' => 'hebi']);
}
public function add_user()
{
// POSTデータを取得
$postData = $this->request->getPost();
return $this->response->setStatusCode(200)->setJSON(['status' => 'success']);
}
}
ルーティングの設定
app/Config/Routes.php ファイルに、POSTリクエストをUserコントローラのadd_userにマッピングします。これによりフロントからadd_userをPOSTで実行できるようになります。
<?php
use CodeIgniter\Router\RouteCollection;
/**
* @var RouteCollection $routes
*/
$routes->get('/', 'Home::index');
// ユーザ取得
$routes->get('user/(:num)', 'User::get_user/$1');
// ユーザ追加
$routes->post('user/', 'User::add_user');
フロントからAPIを実行
ルーティングで指定したuserをurlに指定してPOSTでajaxを実行します。dataにはusername:hebihebiを渡してます。
function addUser(e) {
$.ajax({
url: '/user',
type: 'POST',
dataType: 'json',
data:{
username:'hebihebi'
},
success: function(response) {
appendAlert('成功','success')
},
error: function(xhr, status, error) {
if (xhr.status === 0) {
appendAlert('ネットワークエラーです。','warning')
} else if (xhr.status === 404) {
appendAlert('存在しません。','warning')
}else{
appendAlert('その他サーバーエラーです。','danger')
}
}
});
}
では実際に動かしてみましょう!
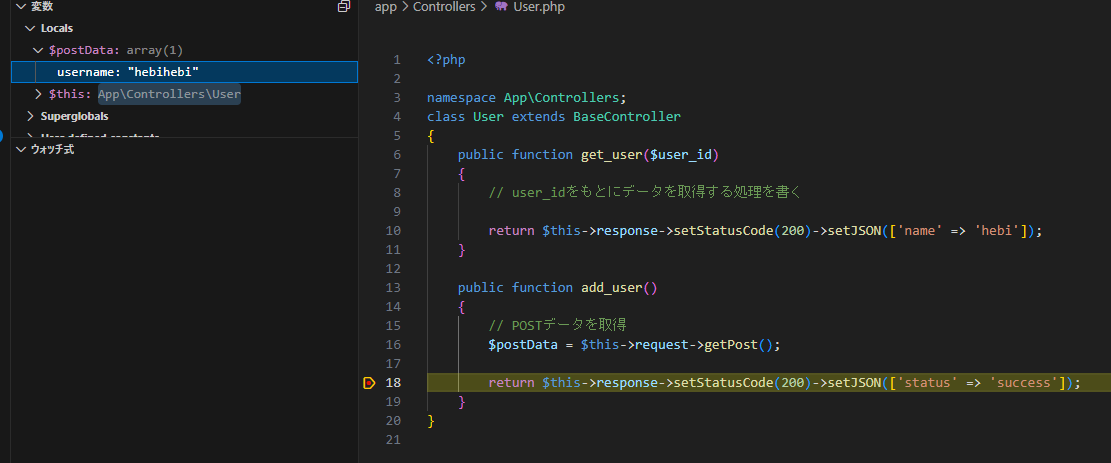
add_userが正常に呼び出され、データも取得できました!
最後に
GETメソッド、POSTメソッドを利用したWeb APIを作成し実行することができたでしょうか?
Web APIをどんどん作成していきましょう!!
最後までお読みいただきありがとうございました(^^)/